The Best Fluffy Pancakes recipe you will fall in love with. Full of tips and tricks to help you make the best pancakes.
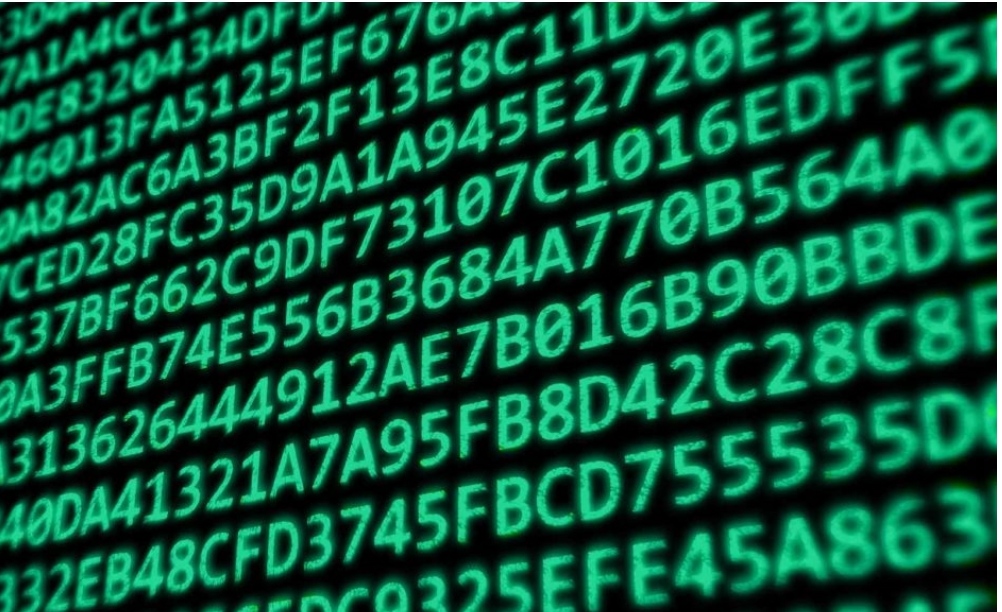
Whirlpool is a cryptographical hash function designed after the discovery of Square block cipher, and it is considered to be part in family of block cipher functions. Whirlpool is a construction based on a substantially modified Advanced Encryption Standard (AES).The Original Whirlpool is called as Whirpool-0.The first version of Whirlpool is called Whirlpool-T.
What is Whirlpool Hash used for?
Whirlpool is a block-cipher-based hash function that used to provide security and performance that is comparable with others. if not better, than that found in non- block-cipher based hash functions, such as SHA( Secure Hash Algorithm).
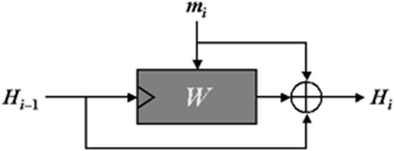
Tricky Question Of Whirlpool
How many rounds are there in one Whirlpool?
There are 4 rounds in each Whirlpool.
How many characters are in the Whirlpool hash?
It will generate 128 characters of Whirlpool hash string and it can not be reversible.
What is the size of message digest in Whirlpool?
The algorithm takes as input a message with a maximum length of less than 2256 bits and produces as output a 512-bit message digest. The input is processed in 512-bit blocks.
What is a major difference between Whirlpool algorithm and SHA?
They found that Whirlpool has a faster computation speed and a lower collision rate than SHA-1.
Whirlpool Hash in Java Script
Whirlpool 512 bit hash in javascript for electron and the browser.
Demo:https://angeal185.github.io/whirlpool-js
Installation:
npm
$ npm install whirlpool-js --save
bower
$ bower install whirlpool-js
git
$ git clone [email protected]:angeal185/whirlpool-js.git
electron
const wp = require('whirlpool-js');
browser
<script src="./dist/whirlpool-js.min.js"></script>
Important terminology
- hex: returns hash as a hex encoded string
- base64: returns hash as a base64 encoded string
- bytes: returns hash as a byte string
- Uint8: returns hash as a Uint8Array
- ArrayBuffer: returns hash as an arraybuffer
API- You can skip the comments. It’s for Your Understand of Code
/**
* sync
* @param {string} str ~ valid string to be hashed
* @param {string} digest ~ hex/base64/Uint8/ArrayBuffer/bytes
**/
wp.encSync(str, digest)
/**
* callback
* @param {string} str ~ valid string to be hashed
* @param {string} digest ~ hex/base64/Uint8/ArrayBuffer/bytes
* @param {function} cb ~ callback function(err,res)
**/
wp.enc(str, digest, cb)
/**
* promise
* @param {string} str ~ valid string to be hashed
* @param {string} digest ~ hex/base64/Uint8/ArrayBuffer/bytes
**/
wp.encP(str, digest)
// demo
const str = 'test';
//sync
let sync = wp.encSync(str, 'hex');
console.log(sync);
//returns hex encoded hash
//callback
wp.enc(str, 'base64', function(err, res){
if(err){return console.log(err)}
console.log(res)
//returns base64 encoded hash
});
//promise
wp.encP(str, 'Unit8').then(function(res){
console.log(res);
//returns Unit8Array of hash
}).catch(function(err){
console.log(err);
}).then(function(){
console.log('done');
})
Whirlpool Hash Function
What is Hash Function?
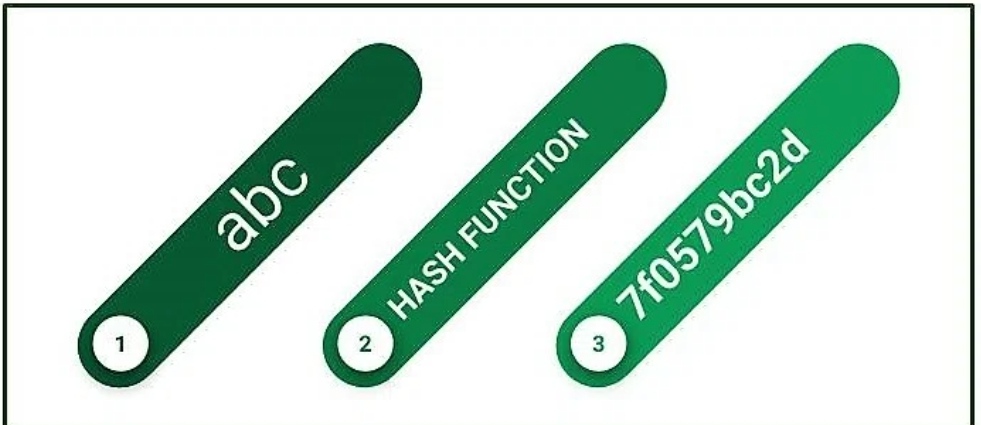
Hash Function is a function which has a huge role in making a System Secure as it converts normal data given to it as an irregular value of fixed length that is hard to predict . We can imagine it to be a mixer in our homes.
When we put data like numbers and alphabets into this function it outputs an irregular value. The Irregular value it outputs is known as “Hash Value”.Hash values are simply number but are written sometimes in Hexadecimal. In Computers stores values as Binary. Hash value is data that is managed as binary in computer.
What is Whirlpool Hash Function?
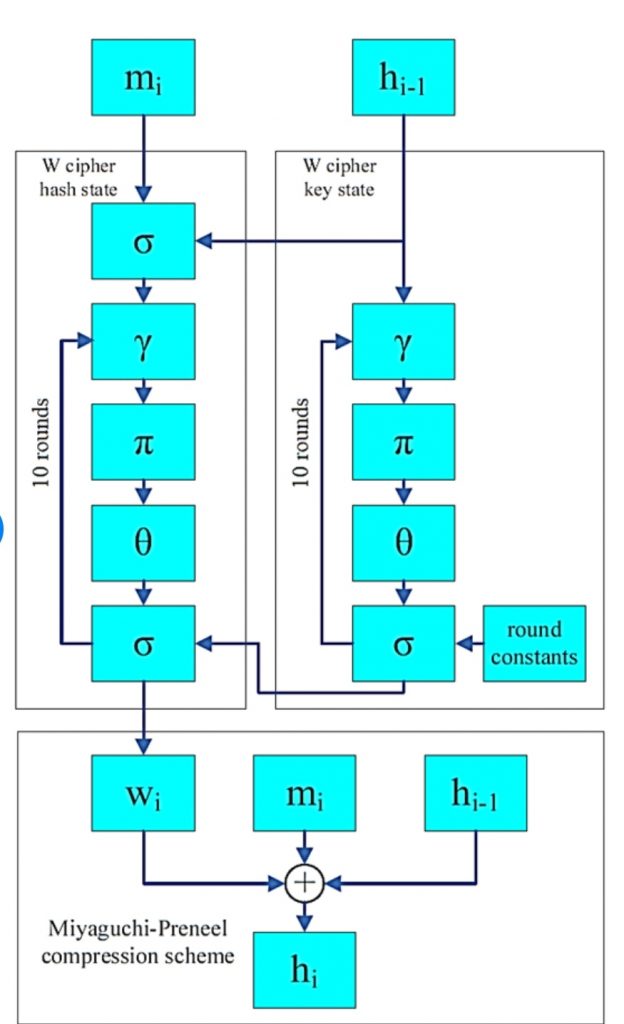
Whirlpool is a cryptographic hashing function created by Vincent Rijmen co-inventor of Advanced Encryption Standerad and paulo S.L.M. Barreto who invented block cipher Anubis . It was first published in year 2000 and revised version of it published in 2001 and 2003 due to copyright issues. It was derived from modified version of square block cipher and Advanced Encryption Standard.
It is a kind of a block cipher hash function that is discovered after square block cipher. It is adopted by International Organization for Standardization (ISO).It takes less than 2^256 bits length input and convert it to return an output of 512 bit hash message .Every block of cipher in whirlpool is a 8*8 rotating matrix constant solved the issue of security created by diffusion matrix. There is an Operation name and its function for specific task –
- Mix Rows(MR)-It is a right multiplication of each row of an 8*8 matrix.
- Substitute Bytes(SB)-It is a simple table lookup and gives nonlinear mapping.
- Add Round Key(AK)-In this the 512 bits of the round key is goes through XOR with 512 bit of current state.
- Shift Columns(SC) -In this except the first column of current state are cyclically downward shifted.
Hash Value is calculated by using the formula:
State = MR*AK*SC*SB(State)
.We are Implementing Using Python.Install whirlpool library using
pip install whirlpool
Example 1- Whirloop Using Python
# Python program to demonstrate
# whirlpool hash function
import whirlpool
string = b"GeeksforGeeks"
h1 = whirlpool.new(string)
hashed_output = h1.hexdigest()
print("The hashed value is")
print(hashed_output)
Output
The hashed value is 95cb4d2d765eb26a922b3ade5a5837a3bc6b18f9a68cec6392f7bf4284c996dd0dd8775dc77964bb9dd92f204d067d3b2c0f36f968607c88cd378ce094438e5a
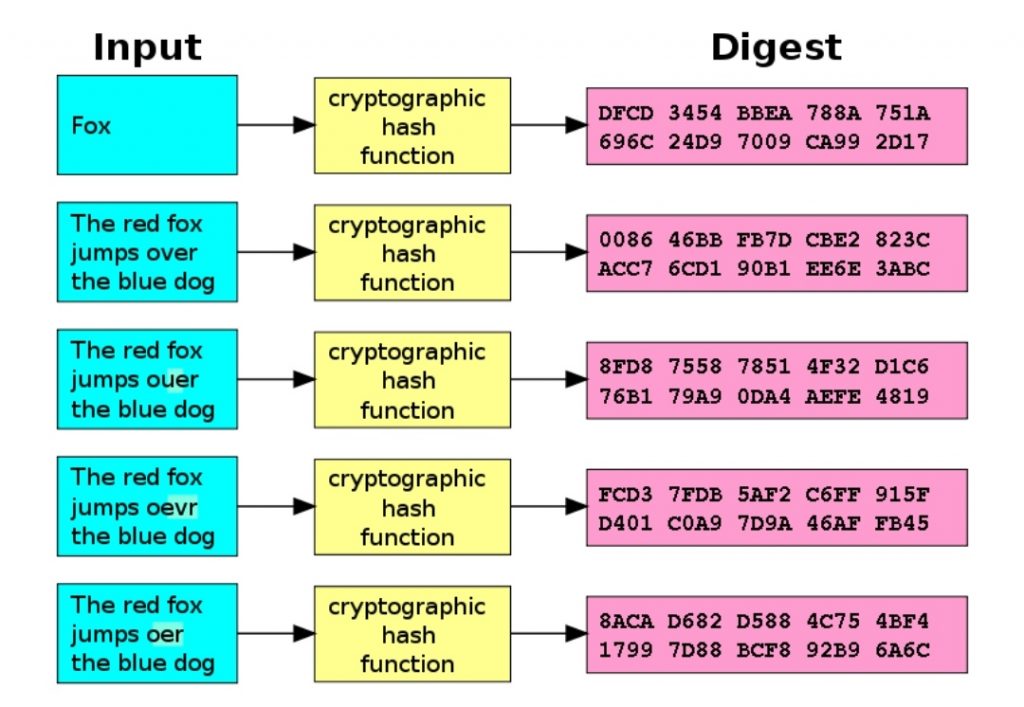
whirlpool.go – A Hashing Library
An opensource Go implement of Whirlpool hashing algorithm. Go is an programming language designed at google . It’s is also called as Golang due to it’s domain name golang.org but it’s Real name is Go.
Setup
$ go get github.com/jzelinskie/whirlpool
Example
package main
import (
"fmt"
"github.com/jzelinskie/whirlpool"
)
func main() {
w := whirlpool.New()
text := []byte("This is an example.")
w.Write(text)
fmt.Println(w.Sum(nil))
}
Is Whirlpool Hash with random salt secure by attack of Hacker?
We have taken a Old website to check it’s vulnerability. We have noticed that password hashing is not up to modern practices. Therefore we have used Salt to secure our password by hackers. The code of it is following:
public function changePW($password){ // $new_salt = $this->random(); $new_password = $this->hash($password, $new_salt); //}
public function random($limit = 10, $from = 32, $to = 126) { $phrase = ''; for ($i=0; $i<$limit; $i++) { $phrase .= chr(rand($from,$to)); } return $phrase;}
// self::$_salt is a constant 12 character string
// of 3 words with some letters replaced by numbers
public static function hash($password, $salt) { return hash('whirlpool', self::$_salt . $password . $salt);}
The salt function shouldn’t use rand()
because it’s not cryptographically secure as per reports.If an attacker knows what the salt is before getting access to the database, it can speed up crack time once access to website resulting advantage for hacker . All salt should be globally unique to make it as much reasonable and secure.
SHA vs MD5 vs Whirlpool
Security hashes are very important to keep user password for various web applications remain secure and safe. SHA, MD5 and Whirloop are encryption available to all web developer without any charge . The question arises
Q which encyrpition method are strongest?
The answer to this is very simple the more bits a hash contains, the more secure web application is from hackers. Among Hashing algorithm MD5 is a 128-bit string, SHA-1 is a 160-bit string and Whirlpool is a 512-bit string if we compare among them whirlpool has highest bit value. .Based on this Whirlpool is clearly superior to both SHA-1 and MD5.There are some reasons why:
- One-way encryption
- Brute Force
- Collisions
- Salt
APPLICATION OF WHIRLPOOL HASHING ALGORITHM
1.Whirlpool Algorithm used in Hash Function Based Watermarking Algorithm for the Secured Transmission of Digital Medical Images.
2.The System on Programmable Chip (SoPC) implementation of the Whirlpool Hash Function (WHF).
3.There is integrated implementation of Whirlpool and AES ( Advance Encyprtion Standered) on FPGA.
I would like to share Python Library used for Whirpool Hashing Function.
https://pypi.org/project/Whirlpool/
Hoping you got all knowledge of Whirpool Algorithm.